Geosetta API Documentation
Welcome to the Geosetta API Documentation. Our platform offers unparalleled access to a wealth of public geotechnical data, empowering your projects with accurate and comprehensive subsurface information. By becoming a sponsor of Geosetta, you not only contribute to the advancement and accessibility of geotechnical data but also gain exclusive API access. This access allows for seamless integration of our extensive data repository into your systems, facilitating more informed decision-making and innovative solutions in your field. Sponsoring Geosetta is more than just a partnership; it's an opportunity to be at the forefront of geotechnical data utilization and to support the ongoing mission of making this valuable data openly accessible to professionals and researchers worldwide. Join us in this endeavor and unlock the full potential of geotechnical data with Geosetta.
API Endpoint to retrive historic Data around Radius:
api_key = "your_api_key_here"
# Your payload and API key
json_payload = {
"deliverableType": "Return_Historic_Data_In_Radius",
"data": {
"points": [
{
"latitude": 39.21884440935613,
"longitude": -76.84346423232522,
"radius_m": 1000
}
]
}
}
# Make the request
response = requests.post(
"https://geosetta.org/web_map/api_key/",
json=json_payload, # Directly send the json_payload
headers={
"Authorization": f"Bearer {api_key}" # Set the API key in the Authorization header
}
)
# Check the response
if response.status_code == 200:
print("API request successful:")
print(response.json())
response_data = response.json() # Convert the Response object to a dictionary
points_in_radius = response_data['results']['points_in_radius']
print(points_in_radius)
else:
print("API request failed:")
print(f"Status code: {response.status_code}")
print(response.text)
The server will respond with a JSON object like this:
{'message': 'Data processed successfully.', 'results': {'points_in_radius': {'type': 'FeatureCollection', 'features': [{'type': 'Point', 'coordinates': [-76.8445259, 39.22082777], 'properties': {'content': ' Geosetta History
\n Source: MDOT
(lat,lng): 39.2208278 , -76.8445259 elev ft: 335.20\n Total Depth: 25.0 ft
Available Deliverables:
\n \n Monthly Precipitation History NOAA
\n \n \n \n
\n View Borehole Log with RSLog\n
\n '}}, {'type': 'Point', 'coordinates': [-76.84413791, 39.22100078], 'properties': {'content': ' Geosetta History
\n Source: MDOT
(lat,lng): 39.2210008 , -76.8441379 elev ft: 346.00\n Total Depth: 5.0 ft
Available Deliverables:
\n \n Monthly Precipitation History NOAA
\n \n \n \n
\n View Borehole Log with RSLog\n
\n '}}, {'type': 'Point', 'coordinates': [-76.8453399, 39.22108078], 'properties': {'content': ' Geosetta History
\n Source: MDOT
(lat,lng): 39.2210808 , -76.8453399 elev ft: 338.10\n Total Depth: 20.0 ft
Available Deliverables:
\n \n Monthly Precipitation History NOAA
\n \n \n \n
\n View Borehole Log with RSLog\n
\n '}}]}}, 'disclaimer': 'The information provided by this API has been made available by various DOTs and other public agencies. The predictive models presented herein are based on machine learning tools applied to the data. The predicted subsurface conditions are a probabilistic model and do not depict the actual conditions at the site. No claim as to the accuracy of the data and predictive model is made or implied. No other representation, expressed or implied, is included or intended and no warranty or guarantee is included or intended in any Geosetta data or models. The User understands these limitations and that Geosetta is a tool for planning subsurface investigations and not a substitute for it. In no event shall Geosetta or the Data Owners be liable to the User or another Party for any incidental, consequential, special, exemplary or indirect damages, lost business profits or lost data arising out of or in any way related to the use of information or data obtained from the Geosetta.org website or API.'}
{'type': 'FeatureCollection', 'features': [{'type': 'Point', 'coordinates': [-76.8445259, 39.22082777], 'properties': {'content': ' Geosetta History
\n Source: MDOT
(lat,lng): 39.2208278 , -76.8445259 elev ft: 335.20\n Total Depth: 25.0 ft
Available Deliverables:
\n \n Monthly Precipitation History NOAA
\n \n \n \n
\n View Borehole Log with RSLog\n
\n '}}, {'type': 'Point', 'coordinates': [-76.84413791, 39.22100078], 'properties': {'content': ' Geosetta History
\n Source: MDOT
(lat,lng): 39.2210008 , -76.8441379 elev ft: 346.00\n Total Depth: 5.0 ft
Available Deliverables:
\n \n Monthly Precipitation History NOAA
\n \n \n \n
\n View Borehole Log with RSLog\n
\n '}}, {'type': 'Point', 'coordinates': [-76.8453399, 39.22108078], 'properties': {'content': ' Geosetta History
\n Source: MDOT
(lat,lng): 39.2210808 , -76.8453399 elev ft: 338.10\n Total Depth: 20.0 ft
Available Deliverables:
\n \n Monthly Precipitation History NOAA
\n \n \n \n
\n View Borehole Log with RSLog\n
\n '}}]}
API Endpoint to retrive data predections (Note you can predect up to 50 points per request with a maximum depth of 100 feet.):
api_key = "your_api_key_here"
# Your payload and API key
json_payload = {
"deliverableType": "Distance_From_Trained_Point",
"data": {
"points": [
{
"latitude": 39.387080,
"longitude": -76.813480,
"depth": 50
},
{
"latitude": 39.4,
"longitude": -76.8,
"depth": 30
}
]
}
}
# Make the request
response = requests.post(
"https://geosetta.org/web_map/api_key/",
json={
"json_data": json.dumps(json_payload) # Encode the dictionary as a JSON string
},
headers={
"Authorization": f"Bearer {api_key}" # Set the API key in the Authorization header
}
)
# Check the response
if response.status_code == 200:
print("API request successful:")
print(response.json())
else:
print("API request failed:")
print(f"Status code: {response.status_code}")
print(response.text)
The server will respond with a JSON object like this:
{'message': 'Data processed successfully.', 'results': {'minimum_distance_from_trained_point_(mi)': 2.13}, 'disclaimer': 'The information provided by this API has been made available by various DOTs and other public agencies. The predictive models presented herein are based on machine learning tools applied to the data. The predicted subsurface conditions are a probabilistic model and do not depict the actual conditions at the site. No claim as to the accuracy of the data and predictive model is made or implied. No other representation, expressed or implied, is included or intended and no warranty or guarantee is included or intended in any Geosetta data or models. The User understands these limitations and that Geosetta is a tool for planning subsurface investigations and not a substitute for it. In no event shall Geosetta or the Data Owners be liable to the User or another Party for any incidental, consequential, special, exemplary or indirect damages, lost business profits or lost data arising out of or in any way related to the use of information or data obtained from the Geosetta.org website or API.'}
This endpoint generates a link to geosetta that will show provided project data alongside public history in geosetta for visualization.
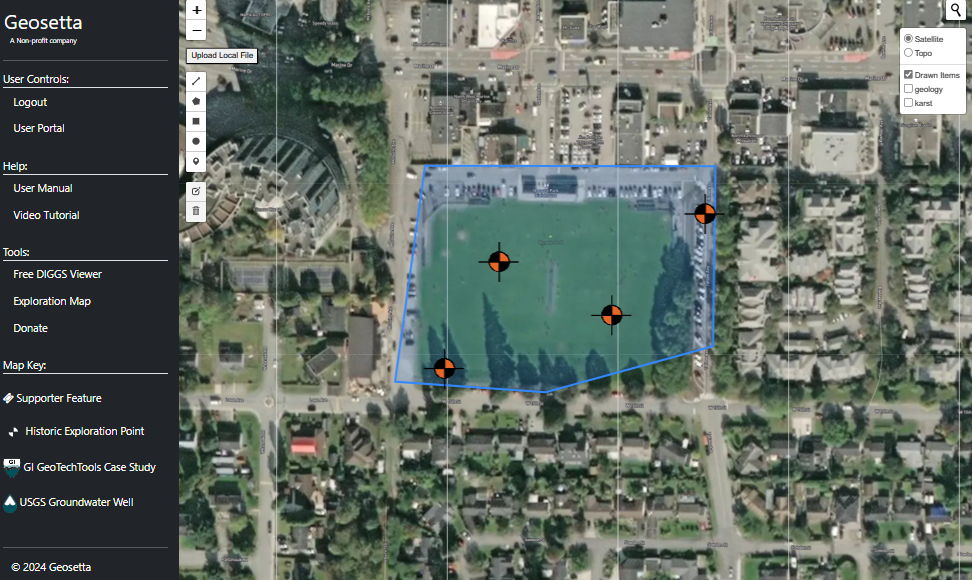
api_key = "your_api_key_here"
# Your payload and API key
json_payload = {
"deliverableType": "Project_Link",
"data": {
"type": "FeatureCollection",
"features": [
{
"type": "Feature",
"geometry": {
"coordinates": [
[
[
-123.119842,
49.323426
],
[
-123.117503,
49.323421
],
[
-123.117524,
49.322477
],
[
-123.118871,
49.322239
],
[
-123.120083,
49.322294
],
[
-123.119842,
49.323426
]
]
],
"type": "Polygon"
},
"properties": {
"Project Title": "RSLog Example Project #1 (Imperial) ",
"Project Number": "EX20-001",
"Client": "ABC Client Company Ltd."
}
},
{
"type": "Feature",
"geometry": {
"coordinates": [
-123.11768,
49.32323
],
"type": "Point"
},
"properties": {
"Name": "AH20-4a",
"Depth": "13 ft",
"Groundwater Depth": "",
"Project Ref.": "RSLog Example Project #1 (Imperial) (EX20-001)"
}
},
{
"type": "Feature",
"geometry": {
"coordinates": [
-123.11934,
49.32298
],
"type": "Point"
},
"properties": {
"Name": "testy",
"Depth": "1 ft",
"Groundwater Depth": "",
"Project Ref.": "RSLog Example Project #1 (Imperial) (EX20-001)"
}
},
{
"type": "Feature",
"geometry": {
"coordinates": [
-123.11843,
49.3227
],
"type": "Point"
},
"properties": {
"Name": "AH21-01",
"Depth": "38.5 ft",
"Groundwater Depth": "",
"Project Ref.": "RSLog Example Project #1 (Imperial) (EX20-001)"
}
},
{
"type": "Feature",
"geometry": {
"coordinates": [
-123.11978,
49.32242
],
"type": "Point"
},
"properties": {
"Name": "AH20-3",
"Depth": "30 ft",
"Groundwater Depth": "16.2 ft",
"Project Ref.": "RSLog Example Project #1 (Imperial) (EX20-001)"
}
}
]
}
}
# Make the request
response = requests.post(
"https://geosetta.org/web_map/api_key/",
json={
"json_data": json.dumps(json_payload) # Encode the dictionary as a JSON string
},
headers={
"Authorization": f"Bearer {api_key}" # Set the API key in the Authorization header
}
)
# Check the response
if response.status_code == 200:
print("API request successful:")
print(response.json())
else:
print("API request failed:")
print(f"Status code: {response.status_code}")
print(response.text)
The server will respond with a JSON object like this:
{'message': 'Data processed successfully.', 'results': 'https://geosetta.org/web_map/map/jqM826', 'disclaimer': 'The information provided by this API has been made available by various DOTs and other public agencies. The predictive models presented herein are based on machine learning tools applied to the data. The predicted subsurface conditions are a probabilistic model and do not depict the actual conditions at the site. No claim as to the accuracy of the data and predictive model is made or implied. No other representation, expressed or implied, is included or intended and no warranty or guarantee is included or intended in any Geosetta data or models. The User understands these limitations and that Geosetta is a tool for planning subsurface investigations and not a substitute for it. In no event shall Geosetta or the Data Owners be liable to the User or another Party for any incidental, consequential, special, exemplary or indirect damages, lost business profits or lost data arising out of or in any way related to the use of information or data obtained from the Geosetta.org website or API.'}
API endpoint retrieves the distance from a pre-trained point. It's important to note that not all data on Geosetta has been included in the training process. Therefore, the distance provided is relative to the nearest location that has been used in our training dataset.
api_key = "your_api_key_here"
# Your payload and API key
json_payload = {
"deliverableType": "Distance_From_Trained_Point",
"data": {
"points": [
{
"latitude": 39.387080,
"longitude": -76.813480,
"depth": 50
},
{
"latitude": 39.4,
"longitude": -76.8,
"depth": 30
}
]
}
}
# Make the request
response = requests.post(
"https://geosetta.org/web_map/api_key/",
json={
"json_data": json.dumps(json_payload) # Encode the dictionary as a JSON string
},
headers={
"Authorization": f"Bearer {api_key}" # Set the API key in the Authorization header
}
)
# Check the response
if response.status_code == 200:
print("API request successful:")
print(response.json())
else:
print("API request failed:")
print(f"Status code: {response.status_code}")
print(response.text)
The server will respond with a JSON object like this:
{'message': 'Data processed successfully.', 'results': {'minimum_distance_from_trained_point_(mi)': 2.13}, 'disclaimer': 'The information provided by this API has been made available by various DOTs and other public agencies. The predictive models presented herein are based on machine learning tools applied to the data. The predicted subsurface conditions are a probabilistic model and do not depict the actual conditions at the site. No claim as to the accuracy of the data and predictive model is made or implied. No other representation, expressed or implied, is included or intended and no warranty or guarantee is included or intended in any Geosetta data or models. The User understands these limitations and that Geosetta is a tool for planning subsurface investigations and not a substitute for it. In no event shall Geosetta or the Data Owners be liable to the User or another Party for any incidental, consequential, special, exemplary or indirect damages, lost business profits or lost data arising out of or in any way related to the use of information or data obtained from the Geosetta.org website or API.'}